Razor is a programming syntax for dynamic ASP.Net web pages released in 2011.
Here’s a C# syntax example from W3 Schools:
<!-- Single statement block -->
@{ var myMessage = "Hello World"; }
<!-- Inline expression or variable -->
<p>The value of myMessage is: @myMessage</p>
<!-- Multi-statement block -->
@{
var greeting = "Welcome to our site!";
var weekDay = DateTime.Now.DayOfWeek;
var greetingMessage = greeting + " Today is: " + weekDay;
}
<p>The greeting is: @greetingMessage</p>
Here’s an example using server-side JavaScript:
<!-- Single statement block -->
<% var myMessage = "Hello World"; %>
<!-- Inline expression or variable -->
<p>The value of myMessage is: <%=myMessage%></p>
<!-- Multi-statement block -->
<%
var greeting = "Welcome to our site!";
var year = new Date().getFullYear();
var greetingMessage = greeting + " The year is: " + year;
%>
<p>The greeting is: <%=greetingMessage%></p>
Eerily similar, eh?
The example above uses server-side JScript hosted in a classic ASP page which is lit up with IntelliSense in VS2012. Classic ASP and JScript were introduced in IIS way back in 1998. With version 3 in 2007 C# introduced the var keyword giving it a similar terse syntax to JavaScript. Nowadays you have the option of hosting classic ASP inside JavaScript itself with Node.js using osASP.
This year I had the privilege of attending and speaking at the Strangeloop conference in St Louis. The venues, the Union Street Marriot (a National Historic Landmark), and the Peabody Opera House were spectacular.
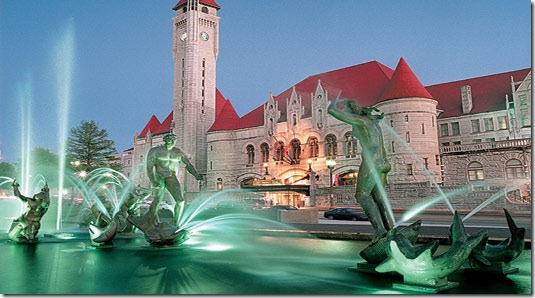
The range of sessions and speakers was literally mind boggling. Some of my favourites:
The breakfast hallway conversations were friendly and insightful, thanks to everyone for all the great feedback on my talk.
F# for Trading
Many people in the community use F# for Trading, and not just developers, traders too. Community matters in a language. The talk looked at some of the reasons people choose to use F# in Trading and some of the projects they have built to support their efforts, like Type Providers for Excel and the R statistical programming language.
The talk was recorded so expect a video to appear on InfoQ at some point in the future.
References
Strangeloop.zip (4.15 mb)
As a C++11 programmer you’ve probably played with type inference and lambda functions and may now feel curious about trying a functional first programming language like F#. F# is a first class .Net programming language that ships with Visual Studio. Although C# shares the familiar moustache based syntax of C++, you may like me actually find F# more familiar in terms of power features like immutability, function inlining and even sprintf .
Comparing some of the good parts of C++11 with C# and F#:
| C++11 | C# | F# |
Architect
| Committee formerly B. Stroustrup | Anders Hejlsberg* | Don Syme |
Statically typed | Yes | Yes
| Yes
|
Generic programming | Template classes, methods & functions e.g. list<T> | Generic types & methods e.g. List<T> | Generic types, methods & functions e.g. list<T> |
Type aliasing | typedef keyword e.g. typedef list<int> xs; | using directive e.g. using xs=List<T>; | type keyword e.g. type xs = list<int> |
Type inference | auto keyword on local & global variables | var keyword on local variables | let keyword on values, variables, parameters & return values |
Math functions | Built-in e.g. abs, min, sin, pow | System.Math class e.g. Math.Abs | Built-in e.g. abs, min, sin, pown |
String format | sprintf function e.g. sprintf(“%d”, 1) | String.Format method String.Format(“{0}”,1) | sprintf function e.g. sprintf “%d” 1 |
Inline functions | inline keyword
| N/A | inline keyword |
Immutability | const correctness mutable keyword | readonly fields | Immutable by default mutable keyword |
Immutable collections | N/A | N/A | Built-in e.g. list, set, map |
Agents | Concurrency runtime Agents library | DevLabs project TPL Dataflow | Built-in MailboxProcessor<T> |
Resource management | Resource acquisition is initialization (RAII) | Explicit scope with using statement on IDisposable objects | Automatic scope with use keyword or explicit scope with using function |
Interop | COM & P/Invoke | P/Invoke & COM | P/Invoke & COM |
* Note: Anders Hejlsberg is reported to be currently working on JavaScript tooling
Resource management
In .Net, garbage collection replaces C++’s delete method for reclaiming memory. For resources like files or database connections that implement IDisposable, .Net can feel a bit more clumsy requiring explicit disposal, over using C++ with RAII.
In C# to determine if a class implements IDisposable you must look at the implementation, and then explicitly dispose the object with either a using block or calling the Dispose method.
using (Font font1 = new Font("Arial", 10.0f))
{
byte charset = font1.GdiCharSet;
}
In F# you can tell if a class implements IDisposable by hovering over it in Visual Studio. Also types implementing IDisposable must be created using the new keyword, which gives an explicit code indication. Finally F# has a use keyword which can be used in place of the let keyword for IDisposable types and automatically calls Dispose when the object is out of scope.
use font1 = new Font("Arial", 10.0f)
let charset = font1.GdiCharSet
Print
Like C++, F# provides a printf and sprintf function. What may be a little more surprising is that in F# they are type safe:

F# will generate a compile time error if the number or type of parameters don’t match.
Headers
Although not required F# supports signatures which are akin to C++ header files and useful for libraries (for example F#’s built-in libraries uses them), particularly for separating code documentation examples from the actual implementation code.
Summary
If you’re interested in augmenting your high performance C++ code with some high level functional code, look beyond the syntax and you may find F# more familiar and feature rich.